First Try
- a tree can be traversed in three different ways
- in this case we try to use pre-order traversal since a tree can be determined as BST based on the BST-ness of its child sub-trees
1 | /** |
The problem with the above solutions is that it doesn’t work for cases like the following where a number smaller than root appears on the right even though the sub-tree is a valid BST.
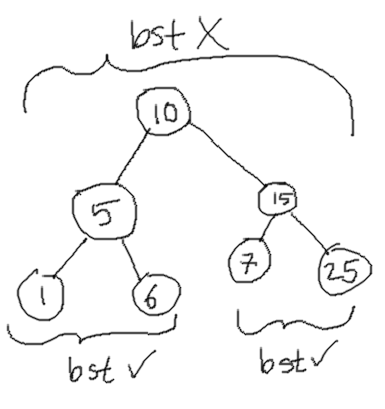
Alternative Approach
Since the approach above is incomplete, let’s think of what a proper BST looks like.
If we perform an inorder traversal of this we see that the numbers are all in ascending order.1
25,50,75,100,125,150,175
So…we can do an inorder traversal and ensure that at any point when we visit a node the last node we visited has the smaller value!
Final solution
1 | /** |
It’s important to keep a track of the pointer since we need to know when the left most node is hit. If we pre-populate with min value such as int prev_val = MIN_INT;
then that will not work in some cases when MIN_INT
is actually a value of the tree. Can you see how?
Tree traversals can be done both using recursive and iterative approaches. Recursive solutions may be more intuitive but they suffer from the possibility of stack overflow. I will plan to have a separate post comparing each of the traversals using both approaches.